ChatGPT API 키 발급받기
1. 브라우저에서 " https://openai.com/ " 사이트에 방문합니다.
2. 계정을 생성합니다.
- 계정은 이메일을 사용하여 가입할 수 있습니다.
- 또한, 구글, 마이크로소프트, 애플 계정을 사용하게 간단하게 생성할 수도 있습니다.
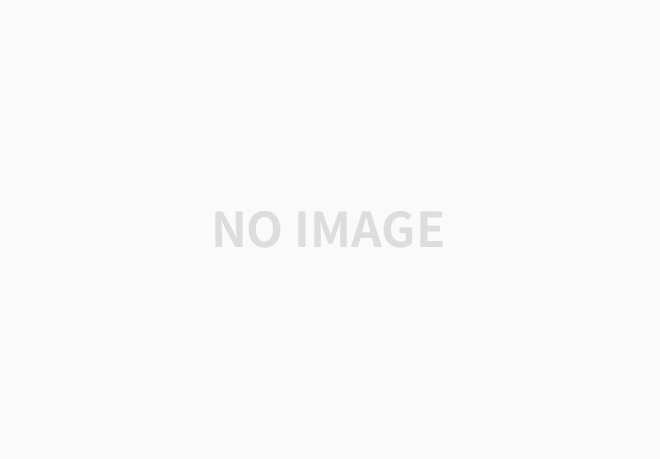
3. API key 생성하기
1). 로그인 후에 OpenAI 웹사이트 우측 상단에 프로필 이미지를 클릭하면 팝업 메뉴가 듭니다.
2). 메뉴에서 View API 키를 선택하면, API 키 관리 화면으로 이동합니다.
3). "Create new secret key" 버튼을 클릭하면 API 키가 발급됩니다.
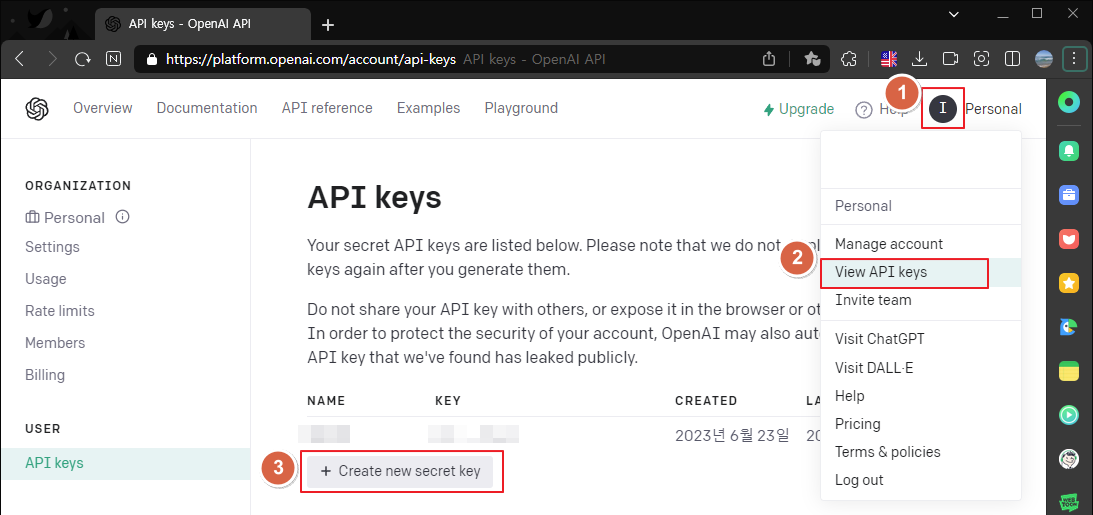
2. C#으로 ChatGPT API사용하기
■ GhatGPT 요청시 필요로 하는것
① openai key
② AI 모델 :
모델설명 (openai 사이트 내용을 번역하였지만, 잘 이해는 안되네요 ^^;;)
GPT-4 | GPT-3.5를 개선하고 자연어(natural language) 또는 코드를 이해하고 생성할 수 있는 모델 세트 |
GPT-3.5 | GPT-3를 개선하고 자연어(natural language) 또는 코드를 이해하고 생성할 수 있는 모델 세트 |
GPT base | 자연어(natural language)나 코드를 이해하고 생성할 수 있는 지시가 없는 모델 집합 |
DALL·E | 자연어(natural language) 프롬프트가 제공되면 이미지를 생성하고 편집할 수 있는 모델 |
Whisper | 오디오를 텍스트로 변환할 수 있는 모델 |
Embeddings | 텍스트를 숫자 형식으로 변환할 수 있는 모델 세트 |
Moderation | 텍스트가 민감하거나 안전하지 않은지 여부를 감지할 수 있는 미세 조정 모델 |
GPT-3 | 자연어를 이해하고 생성할 수 있는 모델 세트 |
ChatGPT-3.5 Turbo는 OpenAI에서 개발한 고급 언어 모델입니다. 인간과 유사한 텍스트를 이해하고 생성할 수 있으므로 챗봇, 콘텐츠 생성 등과 같은 다양한 애플리케이션에 매우 유용한 도구입니다.
③ Randomness: 0과 1 사이 값, 값이 높을수록 무작위 텍스트가 많아집니다.
④ 최대 토큰 값: 높을 수록 많은 분량의 답변을 받을 수 있습니다. (대신 답변이 늦어집니다.)
※ 아래 코드는 코드 프로젝트를 참고하였습니다.
1). 우선 아래와 같은 형태로 디자인을 합니다.
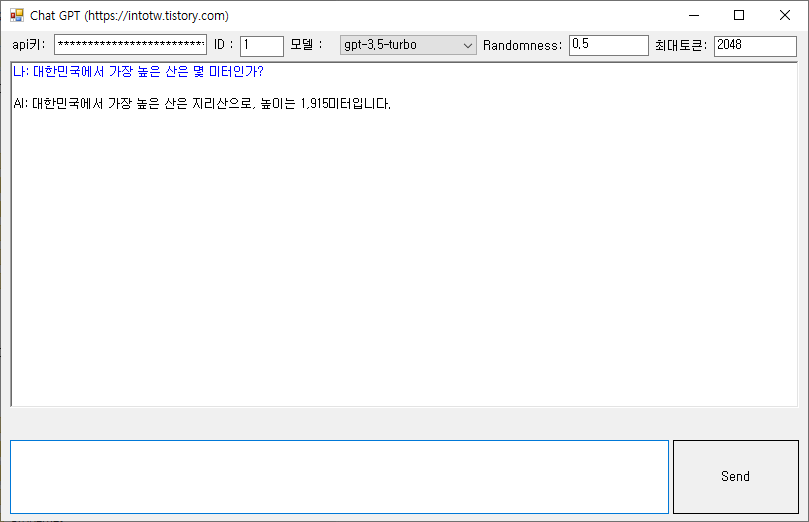
2). 부분 코드는 아래와 같습니다.
◆ 메세지 보내고/ 응답 대기 함수
gpt-3.5-turbo을 사용합니다.
"https://api.openai.com/v1/chat/completions" 이 주소에
chatgpt model, Temperature, Randomness, MaxToken 값을 json 형태로 만들어서 보내고, 응답을 기다립니다.
public string SendMsg(string sQuestion)
{
string sModel = cbModel.Text; // text-davinci-002, text-davinci-003
string sUrl = "https://api.openai.com/v1/completions";
if (sModel.IndexOf("gpt-3.5-turbo") != -1)
{
// gpt-3.5-turbo
sUrl = "https://api.openai.com/v1/chat/completions";
}
var request = WebRequest.Create(sUrl);
request.Method = "POST";
request.ContentType = "application/json";
request.Headers.Add("Authorization", "Bearer " + apikey.Text);
int iMaxTokens = int.Parse(txtMaxTokens.Text); // 2048
double dTemperature = double.Parse(txtTemperature.Text); // 0.5
if (dTemperature < 0d | dTemperature > 1d)
{
MessageBox.Show("Randomness는 0과 1 사이 값, 값이 높을수록 무작위 텍스트가 많아집니다.");
return "";
}
string sUserId = txtUserID.Text; // 1
string data = "";
if (sModel.IndexOf("gpt-3.5-turbo") != -1)
{
data = "{";
data += " \"model\":\"" + sModel + "\",";
data += " \"messages\": [{\"role\": \"user\", \"content\": \"" + PadQuotes(sQuestion) + "\"}]";
data += "}";
}
else
{
data = "{";
data += " \"model\":\"" + sModel + "\",";
data += " \"prompt\": \"" + PadQuotes(sQuestion) + "\",";
data += " \"max_tokens\": " + iMaxTokens + ",";
data += " \"user\": \"" + sUserId + "\", ";
data += " \"temperature\": " + dTemperature + ", ";
data += " \"frequency_penalty\": 0.0" + ", "; // Number between -2.0 and 2.0 Positive value decrease the model's likelihood to repeat the same line verbatim.
data += " \"presence_penalty\": 0.0" + ", "; // Number between -2.0 and 2.0. Positive values increase the model's likelihood to talk about new topics.
data += " \"stop\": [\"#\", \";\"]"; // Up to 4 sequences where the API will stop generating further tokens. The returned text will not contain the stop sequence.
data += "}";
}
using (var streamWriter = new StreamWriter(request.GetRequestStream()))
{
streamWriter.Write(data);
streamWriter.Flush();
streamWriter.Close();
}
var response = request.GetResponse();
var streamReader = new StreamReader(response.GetResponseStream());
string sJson = streamReader.ReadToEnd();
// Return sJson
var oJavaScriptSerializer = new System.Web.Script.Serialization.JavaScriptSerializer();
Dictionary<string, object> oJson = (Dictionary<string, object>)oJavaScriptSerializer.DeserializeObject(sJson);
Object[] oChoices = (Object[])oJson["choices"];
Dictionary<string, object> oChoice = (Dictionary<string, object>)oChoices[0];
string sResponse = "";
if (sModel.IndexOf("gpt-3.5-turbo") != -1)
{
Dictionary<string, object> oMessage = (Dictionary<string, object>)oChoice["message"];
sResponse = (string)oMessage["content"];
}
else
{
sResponse = (string)oChoice["text"];
}
return sResponse;
}
private string PadQuotes(string s)
{
if (s.IndexOf("\\") != -1)
s = s.Replace("\\", @"\\");
if (s.IndexOf("\n\r") != -1)
s = s.Replace("\n\r", @"\n");
if (s.IndexOf("\r") != -1)
s = s.Replace("\r", @"\r");
if (s.IndexOf("\n") != -1)
s = s.Replace("\n", @"\n");
if (s.IndexOf("\t") != -1)
s = s.Replace("\t", @"\t");
if (s.IndexOf("\"") != -1)
return s.Replace("\"", @"""");
else
return s;
}
◆ 실행함수
- 버튼 클릭시 위에서 작성한 메세지를 보내는 함수를 사용하는 쓰레드를 만듭니다.
string OPENAI_API_KEY = "위에서 받은 OPEN AI Key 입력";
// https://beta.openai.com/account/api-keys
public GhatGPT()
{
InitializeComponent();
picLoader.Visible = false;
cbModel.SelectedIndex = 3;
}
private void btnUpdate_Click(object sender, EventArgs e)
{
// apikey.Text 에 openai key 입력시 아래 삭제
apikey.Text = OPENAI_API_KEY;
if (String.IsNullOrEmpty(apikey.Text))
{
txtAnswer.SelectionColor = Color.Red;
txtAnswer.AppendText("[주의]: " + "ChatGPT Api 키를 입력하세요!!!" + "\r\n");
txtAnswer.AppendText("https://platform.openai.com/overview 사이트 가입 후 api키 발급.\r\n");
}
else
{
try
{
Thread threadInput = new Thread(ChatBox);
threadInput.Start();
}
catch (Exception ex)
{
MessageBox.Show("에러: \n\r \n\r" + ex.Message);
}
}
}
private void ChatBox()
{
string sQuestion = txtQuestion.Text;
if (string.IsNullOrEmpty(sQuestion))
{
MessageBox.Show("질문할 내용을 입력하세요!");
txtQuestion.Focus();
return;
}
if (txtAnswer.Text != "")
{
txtAnswer.AppendText("\r\n");
}
txtAnswer.SelectionColor = Color.Blue;
txtAnswer.AppendText("나: " + sQuestion + "\r\n");
txtAnswer.ScrollToCaret();
txtQuestion.Text = "";
btnUpdate.Enabled = false;
//할일
try
{
string sAnswer = SendMsg(sQuestion) + "";
Thread.Sleep(200);
txtAnswer.SelectionColor = Color.Black;
txtAnswer.AppendText("AI: " + sAnswer.Replace("\n", "\r\n").Trim());
txtAnswer.ScrollToCaret();
txtAnswer.AppendText("\r\n");
txtAnswer.ScrollToCaret();
}
catch (Exception ex)
{
txtAnswer.AppendText("Error: " + ex.Message);
}
btnUpdate.Enabled = true;
}
'공학속으로 > C#' 카테고리의 다른 글
C# Naver Api 사용하여 네이버 뉴스 검색하기 (1) | 2023.09.01 |
---|---|
C# 리스트뷰(ListView) 사용하기 (0) | 2023.08.29 |
C# 리스트박스(ListBox) 사용법 (0) | 2023.07.07 |
[C#] 파일 폴더 드래그앤드롭 (1) | 2023.07.07 |
C# ini 사용하기 (0) | 2023.07.06 |
댓글